Objectives:
Using a relatively simple model, simulate a virus outbreak in a domain. Test hypotheses and sensitivities of outbreak spread and rate of infection to a variety of parameters.
- How does air travel affect the speed and extent of a virus outbreak
- How does vaccine development and deployment affect the speed, extent and final numbers of people infected.
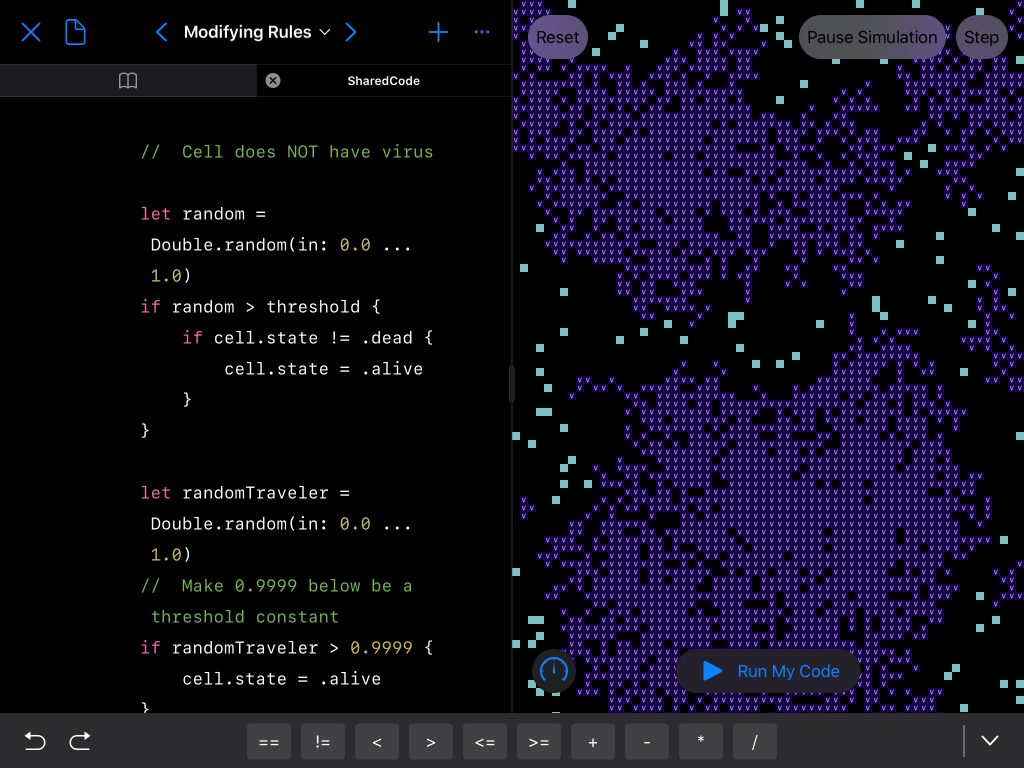
Prior to lesson: Have students work through the “Blink” challenge available on Swift Playgrounds. Take sufficient time to explore the way the model works and have students try out their own rules for making cells alive, dead and idle. Share student’s favorite simulations with each other and discuss the rules associated with each.
As a group discussion, show a few students rules as code. Translate the code to corresponding native language rules. Have all other students predict the resulting simulation’s behavior before running it.
Lesson: First work on modifying the properties of the Simulation in the main program. We changed our cell dimension to be 8 to fit many more cells in the domain. We changed our background colors and characters to more appropriately model a virus, an idle cell, and a vaccinated cell, instead of alive, dead, and idle. And we changed the simulation speed to be 10 to more quickly iterate through the life cycle of the virus outbreak.
Develop the virus simulation in three phases: 1) simulating a virus that spreads both randomly and whose contagion risk depends on the number of surrounding “cells” that are infected; 2) simulating air travel within the domain; 3) simulating the development and distribution of a vaccine.
Watch the video below to see the development of the code in action:
Final state of the configureCell(cell: Cell) function code in the video above:
// Shared Code // Code written in this file is available on all pages in this Playground Book. // ------------------------------------ // Simple Configure Cell Function // ------------------------------------ var vacThreshold = 0.999 public func configureCell(cell: Cell) { let threshold = 1.0 - 0.1 * Double(cell.numberOfAliveNeighbors) switch cell.state { case .alive: // Cell has virus cell.state = .alive case .dead: // Case is used to represent cells that have been vaccinated cell.state = .dead case .idle: let vacRandom = Double.random(in: 0.0 ... 1.0) if vacRandom > vacThreshold { cell.state = .dead } // Cell does NOT have virus let random = Double.random(in: 0.0 ... 1.0) if random > threshold { if cell.state != .dead { cell.state = .alive } } let randomTraveler = Double.random(in: 0.0 ... 1.0) // Make 0.9999 below be a threshold constant if randomTraveler > 0.9999 { cell.state = .alive } } vacThreshold -= 0.0000001 }
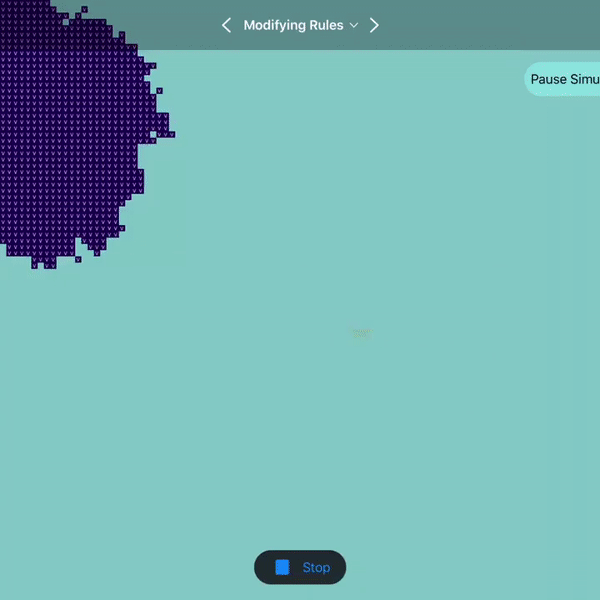
Homework Suggestions: Separate into groups to test a variety of values of three different model parameters: 1) the factor used to multiply by the numberOfAliveNeighbors to determine the influence of neighbors on communicability of the virus; 2) the randomTraveler constant that determines how many cells infect remote locations via air travel; 3) the vacThreshold constant that determines how quickly a vaccine is distributed to the population.
Have the students summarize the impact the values have on the simulations.
Have students estimate what they think is the most correct, or appropriate value for their parameter.
Final Activity: Come together as a group and have a discussion or debate on which parameter is the most important to the simulation. Which parameter has the most impact? Have students journal a plan of action to limit the spread of a virus outbreak as if they were the leader of a large country.
By performing experiments with this simple model you should work toward an understanding that a virus outbreak is a race against time to develop and disseminate a vaccine before the virus spreads too wide to be controllable. The most helpful way to limit the speed of the virus expansion is to limit mobility or remote travel of the population as the virus is spreading.